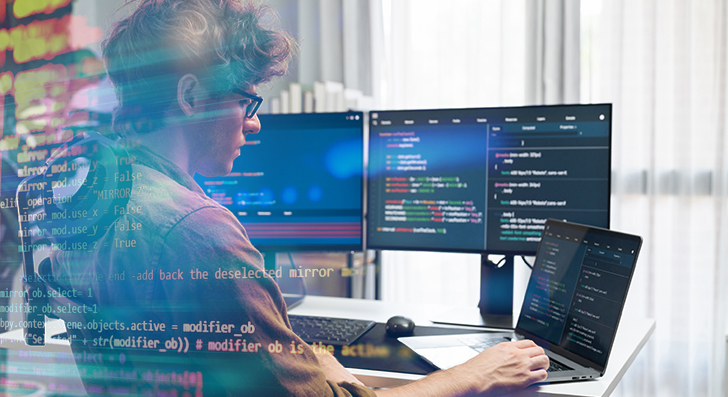
Scalability implies your software can cope with expansion—a lot more customers, more facts, and a lot more targeted traffic—without having breaking. As being a developer, setting up with scalability in mind saves time and strain later. In this article’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it ought to be component within your program from the start. Several purposes fall short every time they increase fast for the reason that the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your system will behave stressed.
Begin by coming up with your architecture to become versatile. Avoid monolithic codebases in which all the things is tightly connected. Alternatively, use modular structure or microservices. These designs crack your app into more compact, unbiased parts. Each and every module or assistance can scale By itself with out impacting the whole program.
Also, contemplate your databases from working day 1. Will it need to have to take care of one million customers or perhaps 100? Pick the correct sort—relational or NoSQL—depending on how your info will expand. System for sharding, indexing, and backups early, Even when you don’t require them nonetheless.
An additional crucial position is to stop hardcoding assumptions. Don’t generate code that only is effective under current situations. Think of what would happen if your user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use structure styles that aid scaling, like information queues or party-driven methods. These assist your app handle more requests without obtaining overloaded.
Once you Construct with scalability in mind, you're not just getting ready for success—you're reducing upcoming problems. A perfectly-prepared program is easier to keep up, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the appropriate Database
Choosing the ideal databases is actually a important Portion of developing scalable applications. Not all databases are designed precisely the same, and using the Completely wrong you can slow you down or simply lead to failures as your application grows.
Begin by understanding your facts. Is it really structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a superb in shape. These are typically robust with interactions, transactions, and consistency. In addition they assist scaling techniques like examine replicas, indexing, and partitioning to deal with additional site visitors and data.
In the event your knowledge is a lot more versatile—like person activity logs, product or service catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling significant volumes of unstructured or semi-structured info and will scale horizontally more simply.
Also, consider your go through and generate patterns. Do you think you're doing a lot of reads with fewer writes? Use caching and read replicas. Will you be managing a hefty publish load? Take a look at databases that may take care of superior create throughput, and even celebration-primarily based facts storage units like Apache Kafka (for temporary info streams).
It’s also sensible to Assume in advance. You may not require Innovative scaling capabilities now, but choosing a database that supports them implies you gained’t need to have to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your information based on your accessibility styles. And generally observe databases general performance when you mature.
To put it briefly, the right databases depends on your application’s composition, speed needs, and how you expect it to grow. Take time to select sensibly—it’ll help save many issues later on.
Optimize Code and Queries
Quick code is vital to scalability. As your app grows, each small hold off provides up. Badly created code or unoptimized queries can slow down general performance and overload your procedure. That’s why it’s essential to Create economical logic from the beginning.
Commence by creating clean, very simple code. Prevent repeating logic and remove something avoidable. Don’t pick the most sophisticated Answer if a straightforward a person will work. Maintain your capabilities small, targeted, and straightforward to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes much too prolonged to run or works by using a lot of memory.
Next, check out your database queries. These generally slow points down over the code alone. Ensure Each and every question only asks for the data you truly require. Prevent Choose *, which fetches anything, and rather pick out particular fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, In particular across huge tables.
For those who discover the exact same data getting asked for again and again, use caching. Retailer the final results temporarily making use of tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database functions any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Make sure to exam with large datasets. Code and queries that function wonderful with one hundred data could possibly crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code limited, your queries lean, and use caching when needed. These steps assist your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and even more website traffic. If all the things goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment aid keep your app speedy, secure, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server undertaking each of the function, the load balancer routes users to different servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out visitors to the Other individuals. Resources like Nginx, HAProxy, or cloud-based alternatives from AWS and Google Cloud make this simple to setup.
Caching is about storing data briefly so it may be reused immediately. When people request the same facts once again—like an item page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it within the cache.
There are 2 common sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents near the user.
Caching lessens database load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust usually. And normally make certain your cache is up-to-date when data does adjust.
To put it briefly, load balancing and caching are straightforward but impressive resources. Collectively, they assist your app manage additional users, remain rapid, and Recuperate from challenges. If you plan get more info to develop, you require both.
Use Cloud and Container Resources
To create scalable purposes, you need resources that allow your application improve easily. That’s exactly where cloud platforms and containers are available in. They provide you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you will need them. You don’t really need to obtain components or guess long run potential. When targeted visitors increases, you can incorporate far more assets with just a couple clicks or routinely employing car-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. You'll be able to give attention to creating your app instead of running infrastructure.
Containers are An additional key Software. A container offers your app and every thing it must operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app concerning environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Resource for this.
Whenever your app works by using a number of containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it routinely.
Containers also allow it to be straightforward to individual parts of your application into solutions. You could update or scale pieces independently, that's great for functionality and reliability.
Briefly, utilizing cloud and container instruments indicates you could scale quickly, deploy conveniently, and Recuperate immediately when difficulties materialize. If you need your application to develop devoid of limits, start off using these instruments early. They conserve time, lessen risk, and enable you to continue to be focused on creating, not repairing.
Monitor Every little thing
When you don’t monitor your application, you gained’t know when matters go Incorrect. Monitoring will help the thing is how your application is carrying out, place issues early, and make far better selections as your application grows. It’s a vital part of creating scalable programs.
Get started by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application far too. Keep an eye on how long it will take for consumers to load webpages, how often problems take place, and the place they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes above a limit or simply a company goes down, you'll want to get notified promptly. This can help you take care of challenges rapid, typically ahead of consumers even discover.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again just before it causes authentic hurt.
As your app grows, targeted visitors and knowledge improve. Without checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, monitoring will help you keep your application dependable and scalable. It’s not almost spotting failures—it’s about knowledge your program and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small applications require a robust foundation. By planning carefully, optimizing properly, and utilizing the right instruments, you can Create applications that develop efficiently without breaking under pressure. Start out small, Feel significant, and Develop sensible.